To provide test data to a test method data provider is used. This allows us to run the same test multiple times with different data sets. This article will show what data provider annotation in TestNG does.
Syntax:
@DataProvider(name = "custom")
How to use:
- Create a method that returns a two-dimensional array of objects. This method will provide the test data for your test method.
- Annotate the data provider method with @DataProvider annotation.
- In the test method, use the @Test annotation with the dataProvider attribute to specify the name of the data provider method.
- Use the test data in your test method by accepting parameters that match the data types in the data provider method.
Example:
package week4.day2;
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
public class DataProviderExample {
@DataProvider(name = "data")
private Object[][] exampleData() {
return new Object[][] { { "Naruto", 26 }, { "Madara", 27 }, { "Sasuke", 40 } };
}
@Test(dataProvider = "data")
private void nameAndAge(String name, int age) {
System.out.println("Name: " + name + ", Age: " + age);
}
}
- In this example, the exampleData() method returns a two-dimensional array of objects containing test data.
- The @DataProvider annotation is used to specify the name of the data provider.
- The nameAndAge() accepts two parameters that match the types of data in the data provider method.
- The @Test annotation is used with the dataProvider attribute to specify the name of the data provider method.
- When you run this test, TestNG will run the nameAndAge() three times, once for each set of data provided by the data provider method.
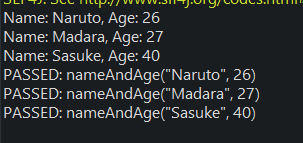
- Log in to post comments