A data-driven framework used in selenium is a fair approach to test software where the test data is separated from the test script. The test data is usually split from here and stored in a separate source, such as an excel or CSV file.
The test script needs to read this data to perform tests.
Why Data-Driven Approach?
- It has more flexibility and reusability of test scripts.
- Changes to the test data can be made without modifying the test script itself.
- It allows for testing multiple data sets, improving the application's coverage under test.
Example:
- This article will demonstrate a data-driven approach using an excel file that contains some login information.
- This login data have username and passwords on a variety of.
- We will use this to log in programsbuzz site.
- One last thing we need is apache POI, a plugin to read/write Microsoft office documents.
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.2.3</version>
</dependency>
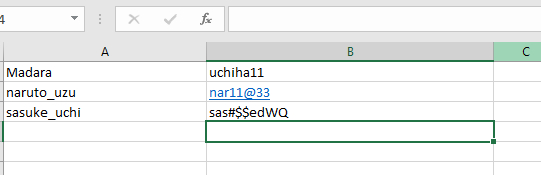
- Here is the excel file with some credentials to test with.
package week4.day2;
import java.io.File;
import java.io.FileInputStream;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelUtil {
XSSFWorkbook work_book;
XSSFSheet sheet;
public ExcelUtil(String excelfilePath) {
try {
File s = new File(excelfilePath);
FileInputStream stream = new FileInputStream(s);
work_book = new XSSFWorkbook(stream);
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
public String getData(int sheet number, int row, int column) {
sheet = work_book.getSheetAt(sheetnumber);
String data = sheet.getRow(row).getCell(column).getStringCellValue();
return data;
}
public int getRowCount(int sheetIndex) {
int row = work_book.getSheetAt(sheetIndex).getLastRowNum();
row = row + 1;
return row;
}
}
- This is an excel read util code.
- This POI library utilizes code that reads the excel and passes in the user name and password.
- It navigates to a specified row and cell of the sheet and fetches the information.
package week4.day2;
import org.openqa.selenium.By;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.DataProvider;
import org.testng.annotations.Test;
import io.github.bonigarcia.wdm.WebDriverManager;
public class ProgramsBuzzUserLogin {
@Test(dataProvider = "testdata")
public void loginPage(String username, String password) {
WebDriverManager.chromedriver().setup();
ChromeDriver driver = new ChromeDriver();
driver.get("https://www.programsbuzz.com/user/login");
driver.manage().window().maximize();
WebElement userName = driver.findElement(By.id("edit-name"));
userName.sendKeys(username);
WebElement passWord = driver.findElement(By.id("edit-pass"));
passWord.sendKeys(password);
}
@DataProvider(name = "testdata")
public Object[][] dataExample() {
ExcelUtil configuration = new ExcelUtil("C:\\Users\\arili\\git\\Assignments\\Selenium\\target\\CRED.xlsx");
int rows = configuration.getRowCount(0);
Object[][] signin_credentials = new Object[rows][2];
for (int i = 0; i < rows; i++) {
signin_credentials[i][0] = configuration.getData(0, i, 0);
signin_credentials[i][1] = configuration.getData(0, i, 1);
}
return signin_credentials;
}
}
- Using the dataprovider annotation, we pass the excelUtil class with the object created for it.
- After passing in the path of the file, we then get its data using a loop.
- Then the signin_credentials are passed onto the username and password field of the programsbuzz site.

- We can see here three tests have run using the following credentials.
- This is how we can create a data-driven framework using selenium java.
- Log in to post comments